Extracting the URL's from Puzzle Rush is time consuming. Using this tool, you only have to open the puzzle URL's.
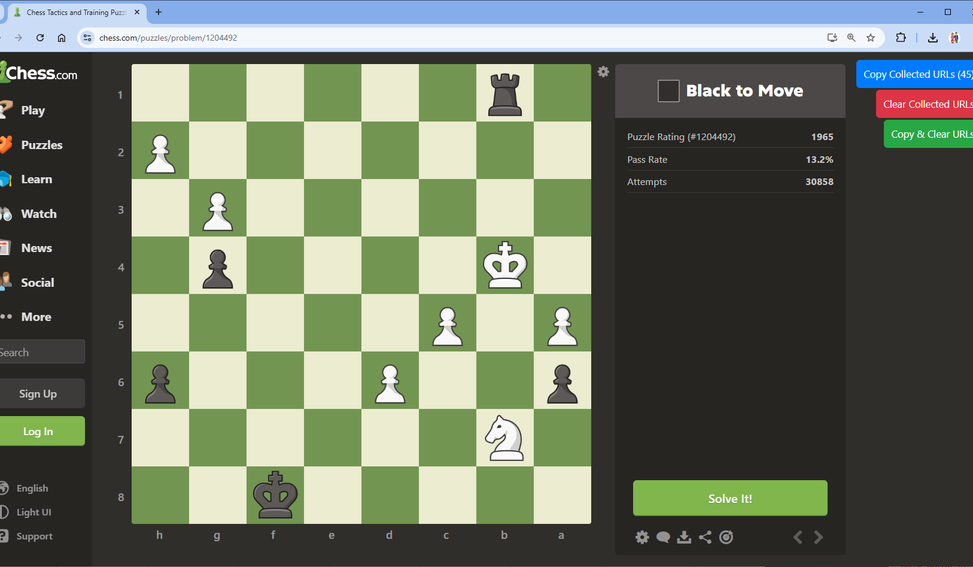
This tool is a tampermonkey script that adds three buttons to the upper right corner of the screen for chess.com. When a puzzle is opened the URL is stored in the browsers memory. To copy the URL's to the clipboard, click Copy Collected URLs.
To use
- Install Tampermonkey extension. Tampermonkey works with all major browsers including Chrome, FIrefox, Edge, Saffari, etc...
- Install the script (below) or by auto install via this link
- Open chess.com and the Blue/Red/Green buttons will appear on the upper right
- Open your chess puzzles
- Only chess puzzle URL's will be stored in memory. We use this tool to collect the failed puzzles so they can be reviewed using the Puzzle Rush Tool
- After all puzzles have been opened, click Copy Collected URLs. This will place all the URL's onto the clipboard.
- Paste the URL's into a spreadsheet or directly into the Puzzle Rush Tool
To clear the list of URL's in memory, click Clear Collected URLs
Installing the script
After Tampermonkey is installed, you can install the script by via this link, or you can manually install it by following the steps below:
After installing Tampermonkey, click on the extensions icon in the upper right and choose Tampermonkey
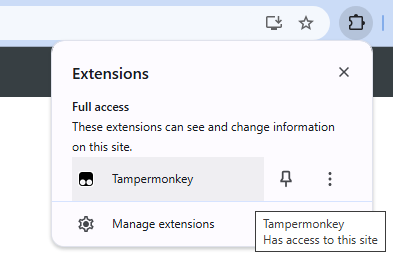
Click on Create a new script...
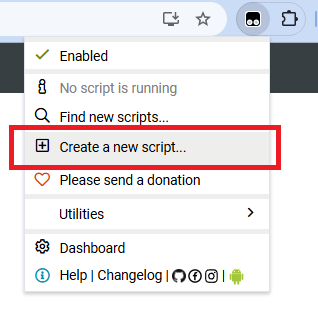
Copy and paste the script below into the edit box
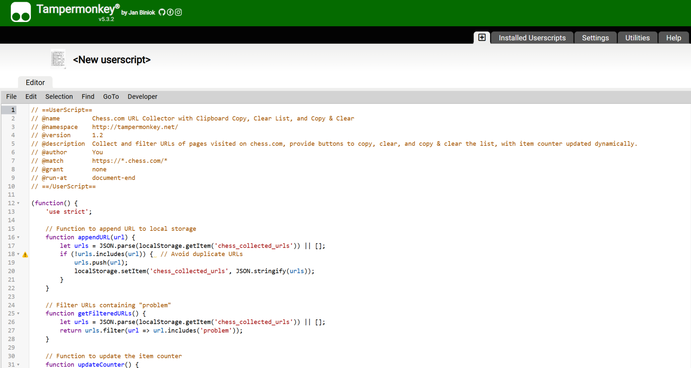
Click File -> Save to save the script
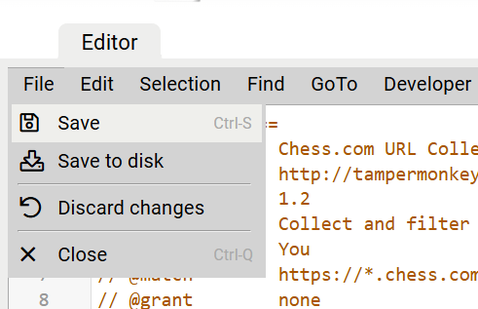
Open chess.com and see the buttons on the upper right side of the screen
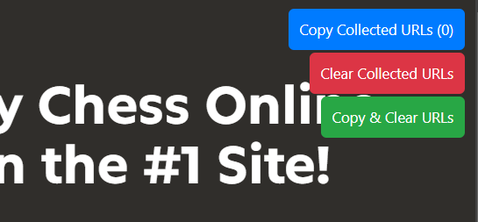
Using the Tool
Login to chess.com and click Stats -> Puzzles.
Scroll to the bottom and click on Rush
Open a puzzle by clicking on one of the rows. It will open in a new window.
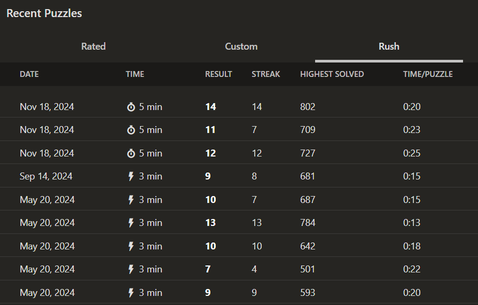
On the puzzle window, click on the red failed puzzle
The puzzle will open in a new window
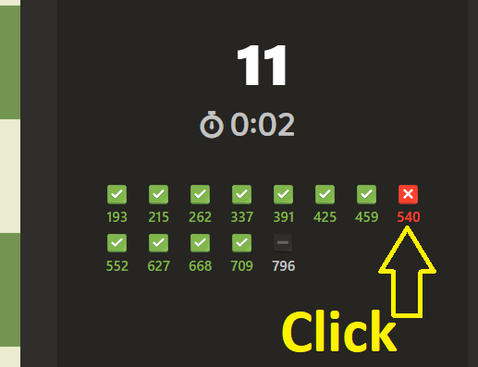
Observe the number of URL's captured has increased to 1
You can close the puzzle window now. The URL's are stored in memory so the puzzle does not need to open.
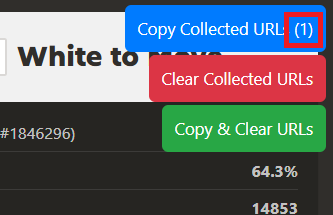
After you have opened all of the URL's, click Copy Collected URLs.
This will place the puzzle URL's on to the clip board.
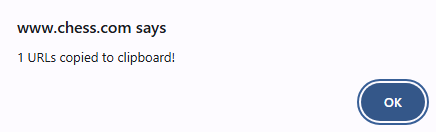
For our studying, we paste the URL's into a google sheet so they can be reviewed in bulk each week.
You can also paste these URL's into the Puzzle Rush Tool which will open each URL so you can retry them.
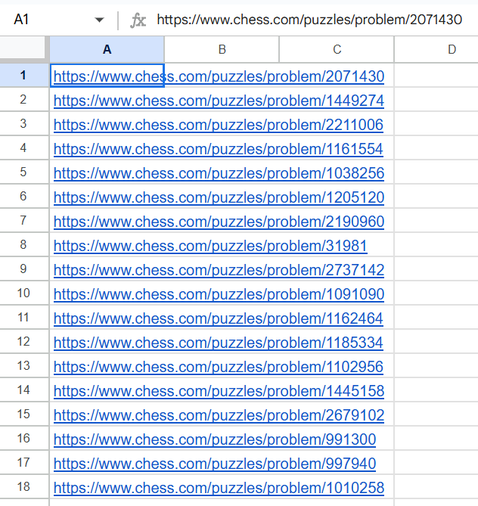
Tampermonkey Script
To install the script, you can either copy/paste the code below, or you can install it from this site: https://greasyfork.org/en/scripts/519629-chess-com-url-collector-with-clipboard-copy-clear-list-and-copy-clear
// ==UserScript==
// @name Chess.com URL Collector with Clipboard Copy, Clear List, and Copy & Clear
// @namespace http://tampermonkey.net/
// @version 1.2
// @description Collect and filter URLs of pages visited on chess.com, provide buttons to copy, clear, and copy & clear the list, with item counter updated dynamically.
// @author You
// @match https://*.chess.com/*
// @grant none
// @run-at document-end
// ==/UserScript==
(function() {
'use strict';
// Function to append URL to local storage
function appendURL(url) {
let urls = JSON.parse(localStorage.getItem('chess_collected_urls')) || [];
if (!urls.includes(url)) { // Avoid duplicate URLs
urls.push(url);
localStorage.setItem('chess_collected_urls', JSON.stringify(urls));
}
}
// Filter URLs containing "problem"
function getFilteredURLs() {
let urls = JSON.parse(localStorage.getItem('chess_collected_urls')) || [];
return urls.filter(url => url.includes('problem'));
}
// Function to update the item counter
function updateCounter() {
let counter = document.querySelector('#chess-url-counter');
if (!counter) return;
let filteredCount = getFilteredURLs().length;
counter.textContent = `(${filteredCount})`;
}
// Function to create the copy button with a counter
function createCopyButton() {
if (document.querySelector('#chess-url-collector-button')) {
return; // Button already exists
}
let button = document.createElement('button');
button.id = 'chess-url-collector-button';
button.innerHTML = 'Copy Collected URLs <span id="chess-url-counter">(0)</span>';
button.style.position = 'fixed';
button.style.top = '10px';
button.style.right = '10px';
button.style.padding = '10px';
button.style.backgroundColor = '#007bff';
button.style.color = '#fff';
button.style.border = 'none';
button.style.borderRadius = '5px';
button.style.cursor = 'pointer';
button.style.zIndex = '9999';
button.addEventListener('click', () => {
let urls = getFilteredURLs();
let urlCount = urls.length;
if (urlCount === 0) {
alert('No URLs matching "problem" to copy.');
return;
}
let urlsString = urls.join('\n');
navigator.clipboard.writeText(urlsString)
.then(() => alert(`${urlCount} URLs copied to clipboard!`))
.catch(err => alert('Failed to copy URLs: ' + err));
});
document.body.appendChild(button);
updateCounter();
}
// Function to create the clear button
function createClearButton() {
if (document.querySelector('#chess-url-clear-button')) {
return; // Button already exists
}
let button = document.createElement('button');
button.id = 'chess-url-clear-button';
button.textContent = 'Clear Collected URLs';
button.style.position = 'fixed';
button.style.top = '50px';
button.style.right = '10px';
button.style.padding = '10px';
button.style.backgroundColor = '#dc3545';
button.style.color = '#fff';
button.style.border = 'none';
button.style.borderRadius = '5px';
button.style.cursor = 'pointer';
button.style.zIndex = '9999';
button.addEventListener('click', () => {
localStorage.removeItem('chess_collected_urls');
alert('Collected URLs have been cleared.');
updateCounter();
});
document.body.appendChild(button);
}
// Function to create the copy and clear button
function createCopyAndClearButton() {
if (document.querySelector('#chess-url-copy-clear-button')) {
return; // Button already exists
}
let button = document.createElement('button');
button.id = 'chess-url-copy-clear-button';
button.textContent = 'Copy & Clear URLs';
button.style.position = 'fixed';
button.style.top = '90px';
button.style.right = '10px';
button.style.padding = '10px';
button.style.backgroundColor = '#28a745';
button.style.color = '#fff';
button.style.border = 'none';
button.style.borderRadius = '5px';
button.style.cursor = 'pointer';
button.style.zIndex = '9999';
button.addEventListener('click', () => {
let urls = getFilteredURLs();
let urlCount = urls.length;
if (urlCount === 0) {
alert('No URLs matching "problem" to copy and clear.');
return;
}
let urlsString = urls.join('\n');
navigator.clipboard.writeText(urlsString)
.then(() => {
localStorage.removeItem('chess_collected_urls');
alert(`${urlCount} URLs copied to clipboard and cleared.`);
updateCounter();
})
.catch(err => alert('Failed to copy URLs: ' + err));
});
document.body.appendChild(button);
}
// Function to ensure buttons are added even if the page content is dynamically loaded
function ensureButtons() {
createCopyButton();
createClearButton();
createCopyAndClearButton();
// Observe DOM changes to handle dynamic content
const observer = new MutationObserver(() => {
if (!document.querySelector('#chess-url-collector-button')) {
createCopyButton();
}
if (!document.querySelector('#chess-url-clear-button')) {
createClearButton();
}
if (!document.querySelector('#chess-url-copy-clear-button')) {
createCopyAndClearButton();
}
});
observer.observe(document.body, { childList: true, subtree: true });
}
// Ensure buttons are created and dynamically update the counter
window.addEventListener('load', () => {
appendURL(window.location.href); // Collect URL on page load
ensureButtons(); // Ensure buttons are added
// Periodically update the counter
setInterval(updateCounter, 2000); // Update every 2 seconds
});
})();
Create Your Own Website With Webador